자바 프로그래밍 입문 강좌 (renew ver.) - 초보부터 개발자 취업까지!!
18. 상속
18-1 상속이란? 부모 클래스를 상속받은 자식 클래스는 부모 클래스의 속성과 기능도 사용할 수 있다.
18-2 필요성
기존의 검증된 class를 이용해 빠르고 쉽게 새로운 class를 만들 수 있다.
18-3 상속 구현
extend 키워드를 이용해 상속을 구현한다.
package pjtTest;
public class ParentClass {
ParentClass() {
System.out.println("ParentClass constrcutor");
}
public void parentFunc() {
System.out.println("parentFunc");
}
}
package pjtTest;
public class ChildClass extends ParentClass{
ChildClass() {
System.out.println("ChildClass constructor");
}
public void childFunc() {
System.out.println("childFunc");
}
}
package pjtTest;
import java.util.Arrays;
import java.util.Scanner;
public class Main {
public static void main(String[] args) {
ChildClass child = new ChildClass();
child.childFunc();
child.parentFunc();
}
}
18-4. 부모 클래스의 private 접근자
자식 클래스는 부모 클래스의 모든 자원을 사용할 수 있지만 private 접근자의 속성과 메서드는 사용할 수 없다.
19. 상속의 특징
19-1. 메서드 오버라이드
부모 클래스의 기능을 자식 클래스에서 재정의하여 사용한다.
package pjtTest;
public class ParentClass {
ParentClass() {
System.out.println("ParentClass constrcutor");
}
public void parentFunc() {
System.out.println("parentFunc");
}
public void makeJjajang() {
System.out.println("--make Jjajang--");
}
}
package pjtTest;
public class ChildClass extends ParentClass{
ChildClass() {
System.out.println("ChildClass constructor");
}
public void childFunc() {
System.out.println("childFunc");
}
@Override
public void makeJjajang() {
System.out.println("--more make Jjajang--");
}
}
package pjtTest;
import java.util.Arrays;
import java.util.Scanner;
public class Main {
public static void main(String[] args) {
ChildClass child = new ChildClass();
child.makeJjajang();
}
}
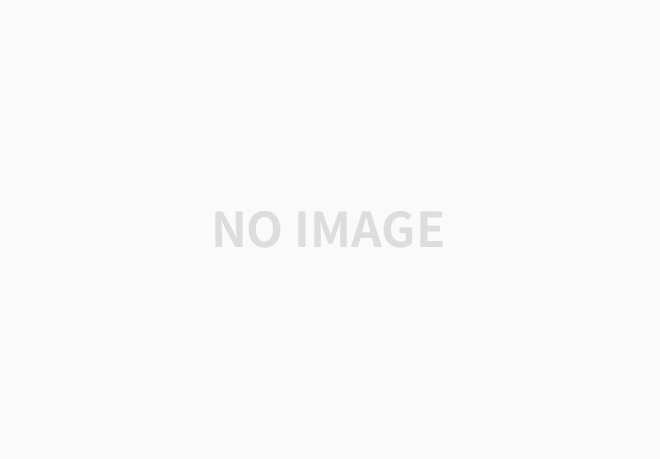
19-2. 기본 자료형처럼 클래스도 자료형이다.
package pjtTest;
import java.util.Arrays;
import java.util.Scanner;
public class Main {
public static void main(String[] args) {
ParentClass childs[] = new ParentClass[2];
childs[0] = new FirstChildClass();
childs[1] = new SecondChildClass();
for(int i =0 ; i<childs.length; i++) {
childs[i].makeJjajang();
}
}
}
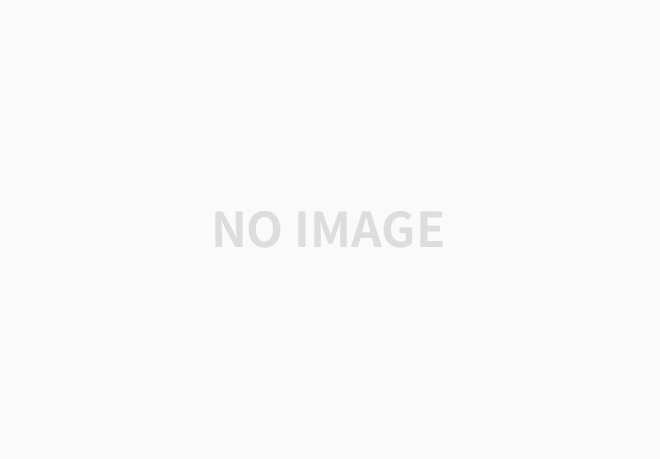
FirstChildClass도 SecondChildClass도 상위형의 데이터 타입이어도 상관없다.
이렇게 지정을 하면 데이터 타입을 통일시켜 같은 배열에 담을 수 있다.
모든 클래스의 최상위 클래스는 Object이다.
19-4. super 클래스
상위 클래스를 호출할 때 super 키워드를 이용한다.
20. 내부 클래스와 익명 클래스
20 -1 내부 클래스
클래스 안에 또 다른 클래스를 선언하는 것으로, 두 클래스의 멤버에 쉽게 접근할 수 있다.
class도 static을 주면 다른 곳에서 바로바로 접근할 수 있다.
package pjtTest;
public class OuterClass {
int num = 10;
String str1 = "java";
static String str11 = "world";
public OuterClass() {
System.out.println("OuterClass constructor");
}
class InnerClass {
int num = 10;
String str2 = str11;
public InnerClass() {
System.out.println("InnerClass constructor");
}
}
static class SInnerClass {
int num = 1000;
String str3 = str11;
public SInnerClass() {
System.out.println("SInnerClass constructor");
}
}
}
package pjtTest;
import java.util.Arrays;
import java.util.Scanner;
public class Main {
public static void main(String[] args) {
OuterClass oc= new OuterClass();
System.out.println("oc.num : " + oc.num);
System.out.println("oc.str11 : " + oc.str11);
OuterClass.InnerClass ic= oc.new InnerClass();
System.out.println("ic.num : " + ic.num);
System.out.println("ic.str11 : " + ic.str2);
OuterClass.SInnerClass si= new OuterClass.SInnerClass();
System.out.println("si.num : " + si.num);
System.out.println("si.str11 : " + si.str3);
}
}
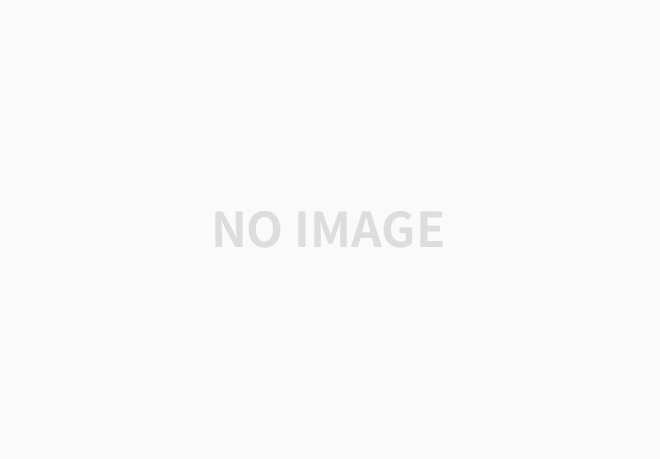
19-2. 익명 클래스
이름이 없는 클래스로 주로 매서드를 재정의 하는 목적으로 사용된다.
public class AnonymousClass {
public void method() {
System.out.println("--Anomymous Class --");
}
}
public static void main(String[] args) {
new AnonymousClass() {
@Override
public void method() {
System.out.println(" --AnonymousClass Override -- ");
};
}.method(); //재정의하자마자 사용
}
객체의 이름이 없다!
21. Interface
21-1.인터페이스란?
클래스와 달리 객체를 생성할 수 없으며, 클래스에서 구현해야 하는 작업 명세서이다.
21-2 사용하는 이유 : 가장 큰 이유는 객체가 다양한 객체가 다양한 자료형을 가질 수 있기 때문이다.
Interface의 데이터 타입을 가질 수 있다.
ex) class가 interfaceA, B, C, D를 implements할 경우, interface A~D까지의 모든 데이터 타입을 가질 수 있다.
public interfaceA {
public void funcA();
}
public class InterfaceClass implements interfaceA {
@OVerride
public void funcA() {
System.out.println("funcA()");
}
}
22. 추상클래스
22-1. 추상클래스란?
클래스의 공통된 부분을 뽑아 별도의 클래스로 만들고, 이것을 상속해서 만든다
- 멤버 변수를 가진다.
- abstract 클래스를 상속하기 위해 extends 사용
- abstract 메서드를 가지며 상속한 클래스에서 구현해야 한다.
- 일반 메소드도 가질 수 있다. 생성자도 있을 수 있다.
package pjtTest;
public abstract class AbstractClassEx {
int num;
String str;
public AbstractClassEx () {
System.out.println(" AbstractClassEx constructor ");
}
public AbstractClassEx (int i, String s) {
System.out.println(" AbstractClassEx constructor2 ");
this.num = i;
this.str = s;
}
public void func1() {
System.out.println("func1()");
}
public abstract void func2();
}
package pjtTest;
public class ClassEx extends AbstractClassEx{
public ClassEx() {
System.out.println("ClassEx constructor");
}
public ClassEx(int i, String s) {
super(i, s);
}
@Override
public void func2() {
System.out.println("func2()");
}
}
package pjtTest;
import java.util.Arrays;
import java.util.Scanner;
public class Main {
public static void main(String[] args) {
AbstractClassEx ex = new ClassEx(10, "java");
ex.func1();
ex.func2();
}
}
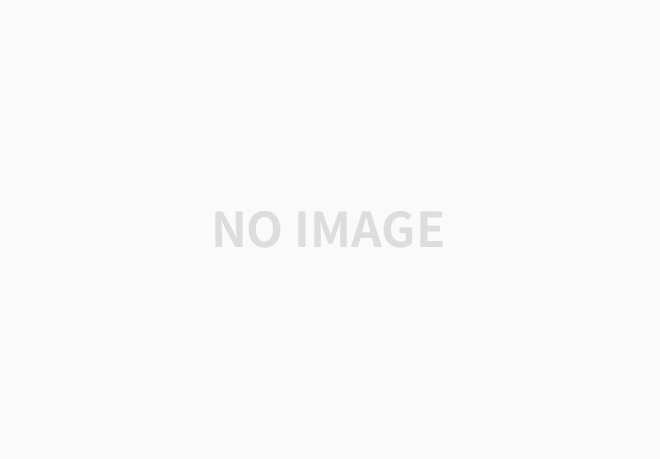
22-4. 인터페이스 vs 추상클래스
공통점 : 추상 메서드 가짐, 객체 생성이 불가하며 자료형으로 사용된다.
인터페이스 : 상수, 추상메서드만 가짐. 추상 메서드를 구현만 하도록 함.
다형성 지원
추상 클래스 : 클래스가 가지는 모든 속성과 기능을 가짐. 단일 상속만 지원!
23. 람다식
23-1. 람다식이란?
익명 함수를 이용해 익명 객체를 생성하기 위한 식이다.
InterfaceType 변수에 implement를 하는게 아니라 interfaceType 변수에 바로 구현하는 것
package pjtTest;
public interface LambdaInterface {
public void method(String s1, String s2, String s3);
}
package pjtTest;
import java.util.Arrays;
import java.util.Scanner;
public class Main {
public static void main(String[] args) {
LambdaInterface li1 = (String s1, String s2, String s3) -> {System.out.println(s1 + " " + s2 + " " + s3); };
li1.method("Hello", "java", "World");
}
}
package pjtTest;
public interface LambdaInterface {
public void method(String s1, String s2, String s3);
}
package pjtTest;
public interface LambdaInterface2 {
public void method(String s);
}
package pjtTest;
public interface LambdaInterface3 {
public void method();
}
package pjtTest;
public interface LambdaInterface4 {
public int method(int x, int y);
}
package pjtTest;
import java.util.Arrays;
import java.util.Scanner;
public class Main {
public static void main(String[] args) {
LambdaInterface li1 = (String s1, String s2, String s3) -> {System.out.println(s1 + " " + s2 + " " + s3); };
li1.method("Hello", "java", "World");
LambdaInterface2 li2 = s1 -> { System.out.println(s1); };
li2.method("Hello");
LambdaInterface3 li3 = () -> System.out.println("haha");
li3.method();
LambdaInterface4 li4 = (x, y)->
{
int result = x*y;
return result;
};
li4.method(10, 20);
li4 = (x, y) -> {
int result = x-y;
return result;
};
li4.method(10, 20);
}
}
24. 문자열 클래스
24-1. String 객체와 메모리
문자열을 다루는 Strinng 객체(클래스)는 데이터가 변하면 메모리 상의 변화가 많아 속도가 느려진다.
문자열이 변경되면 기존의 객체를 버리고 새로운 객체를 메모리에 생성한다.
24-2. StringBuffer, StringBuilder
String 단점을 보완한 클래스로 데이터가 변경되면 기존 데이터를 재사용한다
StringBuffer sf = new StringBuffer("Java");
sf.append("_8";
StringBuilder가 조금 더 빠르며 데이터 안정성은 StringBuffer가 조금 더 좋다.
sf.insert(인덱스, 추가할 문자열); //원하는 인덱스에 문자열 추가 가능
sf.delete(start, end); //인덱스를 지정해 삭제 가능
25. Collections
25-1. List는 인터페이스로 이를 구현한 클래스는 인덱스를 이용해 데이터를 관리한다.
List - Vector, ArrayList, LinkedList.
인덱스를 이용한다. 데이터 중복이 가능하다.
package pjtTest;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.Scanner;
public class Main {
public static void main(String[] args) {
ArrayList<String> list = new ArrayList<String>();
list.add("Hello");
list.add("Java");
list.add("World");
System.out.println(list);
list.add(2, "Programming");
System.out.println(list);
list.set(1, "C");
System.out.println(list);
String str = list.get(2);
System.out.println(list);
str = list.remove(2);
System.out.println(list);
list.clear();
boolean b = list.isEmpty();
System.out.println(b);
}
}
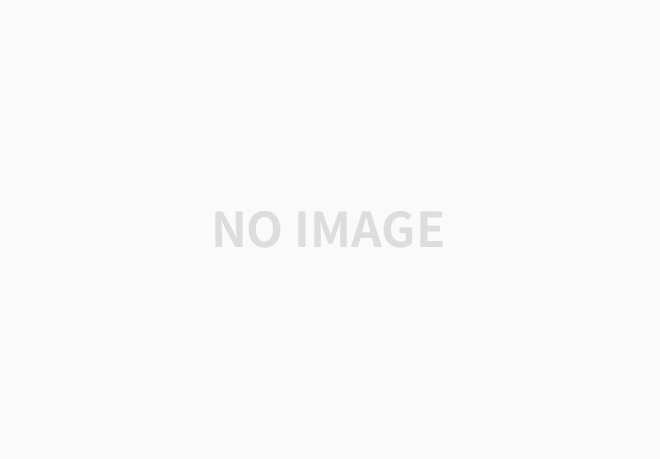
25-2. Map
Key를 이용해 데이터를 관리한다.
Map -> HaspMap (구현)
- key를 이용하며 key는 중복될 수 없다. 중복이 가능하다.
package pjtTest;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.HashMap;
import java.util.Scanner;
public class Main {
public static void main(String[] args) {
HashMap<Integer, String> map = new HashMap<Integer, String>();
System.out.println("map.size() : " + map.size());
map.put(5, "Hello");
map.put(6, "Java");
map.put(7, "World");
System.out.println("map : " + map);
System.out.println("map.size() : " + map.size());
map.put(6, "C");
System.out.println("map : " + map);
String str = map.get(5);
System.out.println(str);
map.remove(7);
System.out.println("map : " + map);
boolean b = map.containsKey(7);
System.out.println(b);
b = map.containsValue("C");
System.out.println(b);
//map.clear()
//map.isEmpty()
}
}
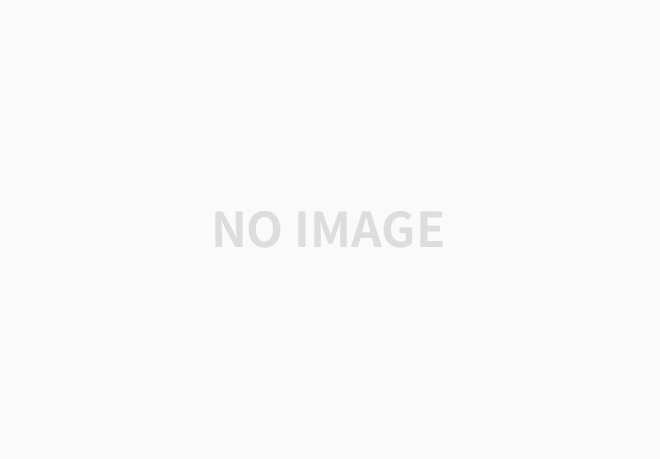
'개발 공부 > Java' 카테고리의 다른 글
자바 프로그래밍 입문 강좌 26~28. JAVA 마무리 (0) | 2020.07.05 |
---|---|
자바 프로그래밍 입문 강좌 11~17. JAVA 객체 (0) | 2020.07.05 |
자바 프로그래밍 입문 강좌 2~10 JAVA 기초 문법 (0) | 2020.07.05 |
(new) 자바 프로그래밍 입문 강좌 1. 오리엔테이션 (0) | 2020.07.05 |